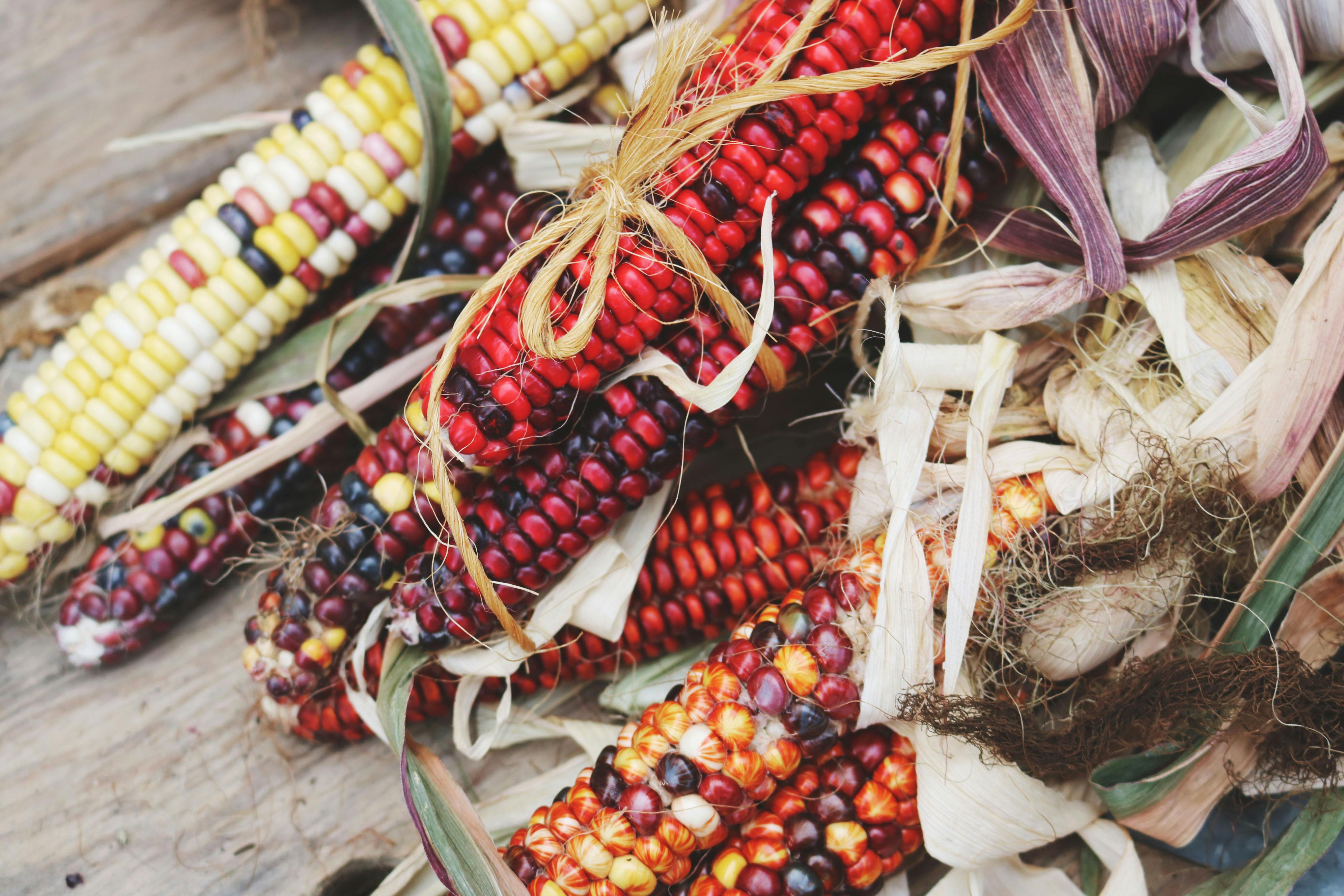
AutoIt – How to copy the contents of a file and paste it using the clipboard
In this tutorial, I show how to quickly copy and paste large amounts of text into form fields after retrieving data from a database table. This is a recent problem that I wanted to solve, as simply sending the variable content to a field takes time if you use Send() and the variable content contains a large amount of text. Just FYI, to use Send() with a variable it is used as follows:
send($variable, 1)
You need the second parameter (ie flag) ‘1’ in the function. The ‘1’ flag means that the data is sent raw. The default value is ‘0’, which is defined as “The text contains special characters such as + and ! to indicate SHIFT and ALT keystrokes.”
AutoIt: ClipPut(), putting content on the clipboard
However, this really isn’t the most efficient method for sending large amounts of text, eg to notepad or form fields. Once you retrieve your data and put this data into a variable, all you need to do is the following:
Func printOutput2()
Local $fTest
$fTest = ClipPut($outputArrayRS[0][2]) ;get value of table's field by index number
Run("notepad.exe")
WinWaitActive("Untitled - Notepad")
Send("^v")
EndFunc
The ClipPut function puts the text into your clipboard. So all you need to do is Send() the Ctrl+v keys like:
send("^v")
AutoIt: ClipGet(), get clipboard content
I tried to use ClipGet function; however this did not work for me. The ClipGet function returns the value when the MsgBox function is used. So with MsgBox() this works:
Func printOutput2()
Local $fTest
$fTest = ClipPut($outputArrayRS[0][2]) ;get value of table's field by index number
MsgBox(0, "test2", ClipGet())
Now to run the function all you need to type is:
printOutput2())
AutoIt: Copy file as in Explorer
If you want to copy a file like you would in Explorer, use the FileCopy function:
FileCopy("C:file_to_copy.txt", "D:mydirfile_to_copy.txt")
The format to use this function is:
FileCopy ( "source", "dest" [, flag] )
There are a few flags you can use with the FileCopy function:
[optional] this flag is a combination or one of the following:
0 = (default) do not overwrite existing files
1 = overwrite existing files
8 = Create destination directory structure if it does not exist.
If you want to combine the flags, add the values. In other words, if you want to overwrite an existing file in the destination and check if the destination directory exists or not, and if not, create the directory, then you would use ‘9’ as the flag.
So the flag would be used as:
FileCopy("C:file_to_copy.txt", "D:mydirfile_to_copy.txt", 9)
You can use wildcards when copying files such as ‘*’ which denotes zero or more characters and ‘?’ denoting zero or one character. So if you wanted to copy all the files to the temporary directory (ie C:temp) and then copy them to another directory, you would use the following code:
FileCopy("C:temp*.*", "D:mydir")
Note that you cannot have more than one wildcard in the file name and extension. So this will NOT work:
FileCopy("C:tempd*g?.*", "D:mydir")
You will need to use regular expressions to accomplish this more complex task.
Another note that applies specifically to the extension is that matches will be made for the first 3 characters of the extension. In other words, this:
FileCopy("C:temp*.txt", "D:mydir")
will copy all files with the extension ‘txt’, as well as any extensions such as ‘txt*’. So the extensions ‘txt1’, ‘txt2’, ‘txt3’ will also be copied.